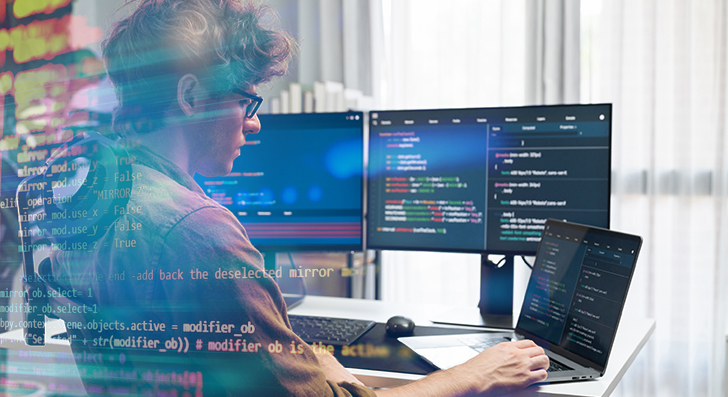
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—without having breaking. As being a developer, setting up with scalability in mind saves time and tension afterwards. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability is just not anything you bolt on later—it should be part of the plan from the start. Many purposes fall short every time they increase fast due to the fact the original layout can’t handle the extra load. To be a developer, you'll want to Believe early regarding how your system will behave under pressure.
Start out by creating your architecture to get adaptable. Stay away from monolithic codebases where almost everything is tightly related. Rather, use modular style and design or microservices. These styles break your app into lesser, independent elements. Each module or support can scale By itself without the need of affecting The entire technique.
Also, contemplate your databases from day 1. Will it have to have to handle a million end users or merely 100? Pick the correct sort—relational or NoSQL—based upon how your details will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t generate code that only works beneath recent problems. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design styles that aid scaling, like information queues or party-pushed programs. These support your app manage a lot more requests devoid of finding overloaded.
Any time you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing foreseeable future complications. A properly-planned method is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Databases
Deciding on the appropriate database is a vital Section of creating scalable applications. Not all databases are crafted the exact same, and using the Improper you can sluggish you down or perhaps cause failures as your application grows.
Begin by being familiar with your knowledge. Is it really structured, like rows in the table? If yes, a relational databases like PostgreSQL or MySQL is a good in good shape. These are generally powerful with interactions, transactions, and consistency. In addition they help scaling techniques like browse replicas, indexing, and partitioning to manage more website traffic and information.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or documents—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with big volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, take into account your read through and write patterns. Will you be doing a great deal of reads with much less writes? Use caching and read replicas. Have you been dealing with a major create load? Look into databases that will cope with high compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also clever to Imagine forward. You may not need to have Sophisticated scaling attributes now, but deciding on a databases that supports them means you won’t require to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based upon your entry styles. And generally keep track of database overall performance while you increase.
In a nutshell, the appropriate databases will depend on your application’s composition, velocity wants, And exactly how you expect it to grow. Take time to pick wisely—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Speedy code is vital to scalability. As your app grows, each little delay provides up. Improperly penned code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s imperative that you Make efficient logic from the beginning.
Start off by composing thoroughly clean, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most elaborate Option if an easy one particular operates. Keep your capabilities limited, targeted, and straightforward to check. Use profiling resources to uncover bottlenecks—destinations in which your code requires as well extensive to run or uses far too much memory.
Following, take a look at your databases queries. These often sluggish things down more than the code by itself. Be certain Just about every query only asks for the info you actually have to have. Keep away from SELECT *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And stay away from doing a lot of joins, Specifically throughout huge tables.
When you discover a similar info remaining requested over and over, use caching. Retail outlet the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and can make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash every time they have to take care of 1 million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software keep clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and much more site visitors. If almost everything goes by a person server, it will speedily turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments enable keep the application rapidly, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout numerous servers. As an alternative to a person server doing each of the do the job, the load balancer routes people to unique servers determined by availability. This suggests no single server gets overloaded. If a person server goes down, the load balancer can mail visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this very easy to create.
Caching is about storing info temporarily so it might be reused speedily. When consumers request a similar facts once again—like a product web page or a profile—you don’t should fetch it from your database anytime. You'll be able to provide it with the cache.
There are two popular forms of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid obtain.
2. Shopper-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching reduces databases load, improves pace, and makes your application more successful.
Use caching for things that don’t adjust usually. And normally ensure your cache is current when information does transform.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application deal with additional customers, keep quickly, and Get better from issues. If you intend to grow, you may need both.
Use Cloud and Container Resources
To develop scalable purposes, you'll need equipment that allow your application grow very easily. That’s wherever cloud platforms and containers come in. They provide you adaptability, reduce setup time, and more info make scaling Considerably smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and expert services as you would like them. You don’t have to purchase hardware or guess potential ability. When website traffic improves, it is possible to insert extra resources with just a few clicks or immediately utilizing automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You may center on constructing your app in lieu of running infrastructure.
Containers are A different critical Device. A container deals your app and everything it really should operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If one particular section of your respective app crashes, it restarts it automatically.
Containers also help it become simple to separate portions of your app into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container applications implies you can scale rapidly, deploy effortlessly, and Get well rapidly when challenges occur. If you prefer your app to improve with out limits, start off applying these equipment early. They help you save time, minimize possibility, and assist you to keep focused on creating, not correcting.
Monitor Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking helps you see how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a critical Element of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for users to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes over a limit or maybe a assistance goes down, it is best to get notified quickly. This will help you correct troubles quickly, usually prior to users even notice.
Checking can be valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it again just before it leads to serious problems.
As your application grows, site visitors and information maximize. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you stay on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Final Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the suitable tools, you may build applications that mature easily devoid of breaking under pressure. Commence compact, Believe major, and build wise.